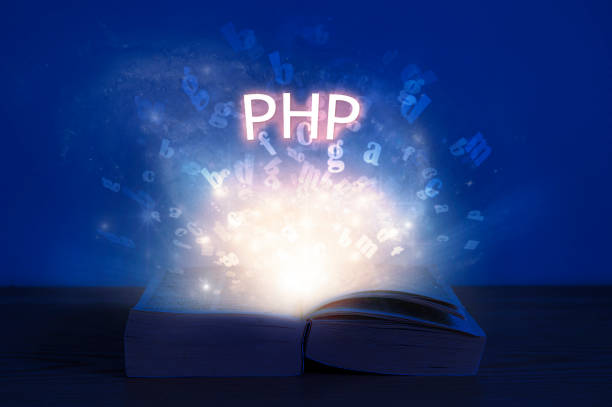
Variables are the backbone of coding. They store information that your code will use to run, make logical decisions with, and even display data to users when you’re developing UI elements. These variables can range from simple numbers like 1 – 10, or complex arrays that could contain all the names of a teacher’s class roster and their grades. While the data that variables store is important, it is also imperative that the way you name your variables is clear and readable so that other coders and even yourself, can understand the meaning of your program without much guesswork.
First off, what is a variable? Variables can be one of eight data types.
- String
- Integer
- Float
- Boolean
- Array
- Object
- Null
- Resource
Each of these 8 types serves a different purpose and can be used to pass different data back and forth. They also will allow you to trigger different logic paths in your code so that it can work the way that you intend. Variables are also used to declare an instance of one of these data types so that you can use it multiple times in your code without having to redeclare the logic every time. This will save you time while coding and make your code easier to read.
Here is an example:
//Adding days that someone worked to a running total
$daysWorkedThisMonth = 15; //Integer
$dailyPayRate = 150.00; //Float
$totalDaysWorkedThisYear = 0;
$totalDaysWorkedThisYear = $totalDaysWorkedThisYear + $daysWorkedThisMonth;
$yearlyPay = $totalDaysWorkedThisYear * $dailyPayRate;
The first thing that you’ll probably notice is that all of these variables start with a “$” and then the name of your variable. That is because in the PHP language, you declare a variable with a “$.” In this example we declare three different variables at the top. They store simple information like the total days that someone worked in a month and how much they get paid per day. You probably were able to figure out what data they were storing based on the name. That is due to the naming conventions and how descriptive we are being with our variables. This will help in the future as our code gets more complex and we start to add even more variables and logic to our program.
Rules for PHP variables:
- A variable starts with the
$
sign, followed by the name of the variable - A variable name must start with a letter or the underscore character
- A variable name cannot start with a number
- A variable name can only contain alpha-numeric characters and underscores (A-z, 0-9, and _ )
- Variable names are case-sensitive (
$age
and$AGE
are two different variables)
Following these rules is a must when working with the PHP language. If you don’t follow these rules, errors will be thrown letting you know that your variable naming is not correct and you will be required to change whichever of these rules you have broken.
Another thing to consider is the way you capitalize certain letters in your variables. The example above uses a convention called Camel Case. This means that the first letter of the first word is lowercase and then the first letter of each other word in the same variable name is capitalized.
$thisIsACamelCaseVariable = true; //Camel Case
$ThISisNoTACameLCaseVariABLE = false; // Not Camel Case
There are a variety of other variable naming conventions similar to camel case naming. Camel case naming is the most commonly used convention but, it may depend on your own choices or the company you work for may specify that you need to use a specific convention. These conventions exist to make your code easier to read for both yourself and other developers.
The biggest reason to think about the names you use for your variables is because in the future someone else may have to read and update your code. It might be easy for you to remember that the variable “$a” stands for the String “apple” but, to someone reading your code in the future this may not be so easy to see for them. The length of your code will also lead variables needing clean and easy to understand names. If you have 1000 lines of code of more, you don’t want to constantly digging through the code to figure out what data a variable contains that you created 10+ hours ago.